Generic Interface
The pyiron IDE is built on top of an abstract class of Python objects, which can be combined like building blocks.
So switching from density functional theory (VASP, SPHInX) to interatomic potentials (LAMMPS) is as easy as changing a variable.
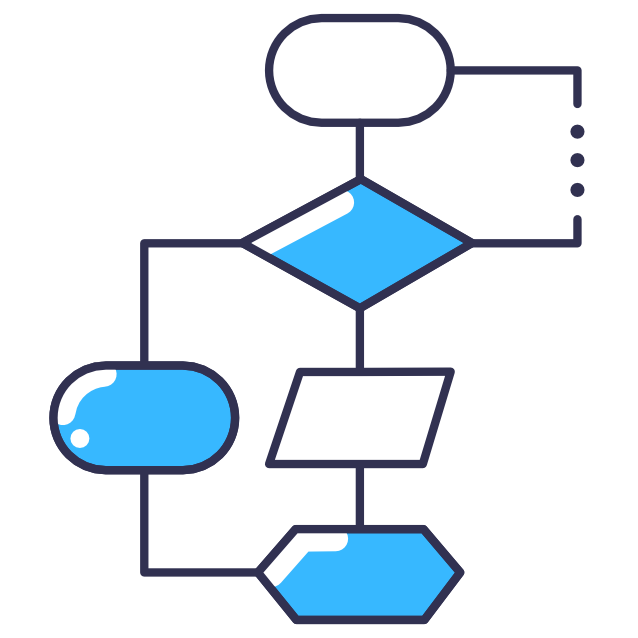
from pyiron import Project # Import Project object
pr = Project("demonstration") # Create a project/folder
structure = pr.create.structure.bulk("Al") # Create an aluminium bulk structure
for job_type in ["Gpaw", "Lammps", "Sphinx"]: # Iterate over simulation codes
job = pr.create_job( # Create a job object
job_type=job_type,
job_name=job_type
)
job.structure = structure # assign the structure
# job.server.queue = "my_queue" # uncomment to up-scale to HPC
# job.server.cores = 4 # Set number of cores
job.run() # Execute the calculation
Up-scaling
To up-scale the interactive calculation to HPC pyiron implements the server object. This enables studying trends e.g. in the periodic table.
The resulting large datasets can be quickly analyzed using the map-reduce pattern: The pyironTable object aggregates the results in a single pandas Dataframe.
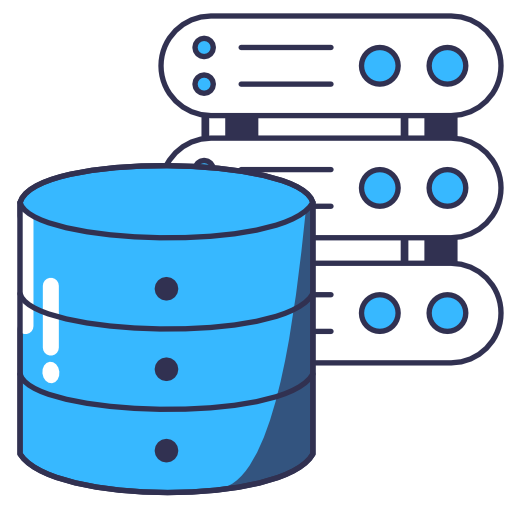
table = pr.create_table() # Create analysis object
table.add.get_energy_tot # Define analysis functions
table.add.get_volume # get the volume and total energy
table.add["job_type"] = lambda job: job.__name__ # Add custom analysis function
table.run() # Execute the analysis
print(table.get_dataframe()) # Results are summarized in DataFrame
Extendability
New simulation codes can be added easily to the pyiron IDE either by using the Python bindings (see example below) or by defining a write_input
and a collect_output
function to parse the input and output files of the executable.
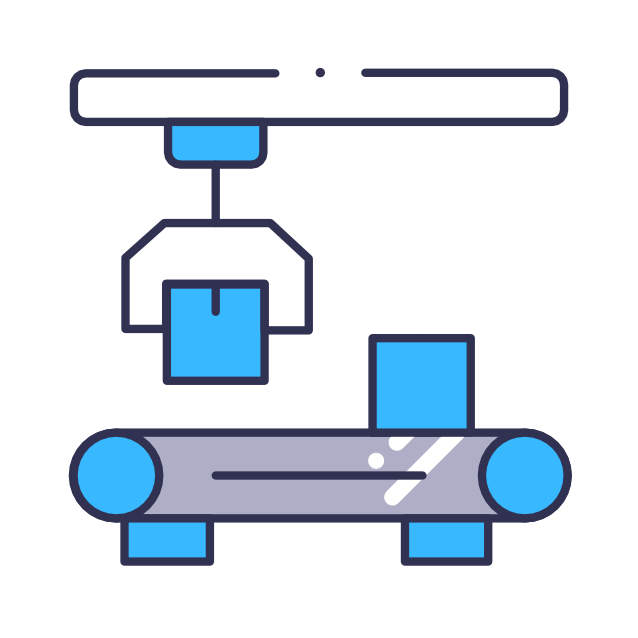
from pyiron_base import PythonTemplateJob
class ToyJob(PythonTemplateJob): # Create a custom job class
def __init__(self, project, job_name):
super().__init__(project, job_name)
self.input.energy = 100 # Define default input
def run_static(self): # Call a python function and store stuff in the output
self.output.double = self.input.energy * 2
self.status.finished = True
self.to_hdf()
job = pr.create_job(job_type=ToyJob, job_name="toy") # Create job instance
job.run() # Execute Custom job class
Latest News
Supercharge your research with reproducibility.